Learning a new programming language is like diving into a new universe. For many newcomers to C#, it’s an exciting journey with lots of potential. But, as with any adventure, there are obstacles along the way. Some challenges are universal to coding, while others are unique to C# and its extensive ecosystem. In this article, we’ll explore the most common hurdles that new C# programmers face and, more importantly, how to overcome them.
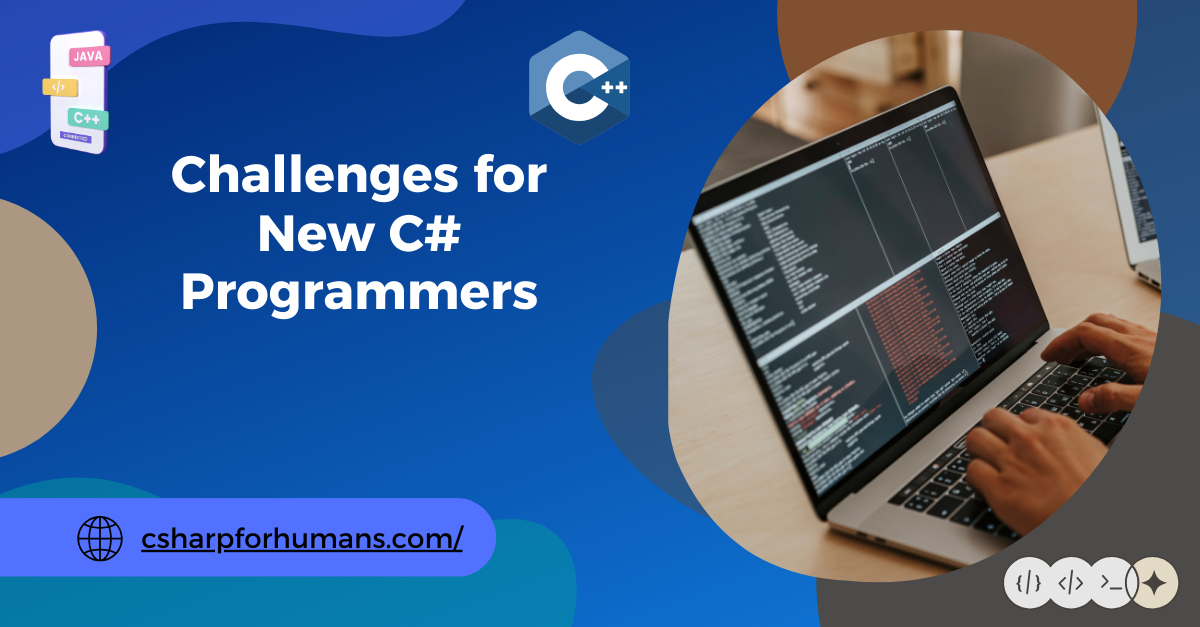
Why Is C# a Popular Choice for Beginners?
C# is known for its readability, versatility, and powerful integration with the .NET framework. It's used across various fields—from game development to web applications. But while C# is beginner-friendly, its deep set of features and robust framework can also create steep learning curves.
Benefits of Learning C#
- Cross-Platform Capabilities: With .NET Core, C# applications can run on Windows, Linux, and macOS.
- Great Community Support: There’s a vast array of tutorials, forums, and documentation to help.
- Object-Oriented Structure: C# is based on OOP principles, making code easier to organize and understand.
Top Challenges for New C# Programmers
1. Understanding the Syntax and Structure
C# syntax can be intimidating for beginners, especially if they’re unfamiliar with programming. The language is strict with its rules and requires specific syntax.
Tips to Master C# Syntax
- Practice Basic Code Daily: Start with simple exercises to get comfortable.
- Use Visual Studio IntelliSense: This feature can correct and suggest syntax as you type.
2. Grasping Object-Oriented Programming (OOP)
C# is deeply rooted in OOP, which can be confusing if you’ve never worked with classes, inheritance, and polymorphism. These concepts require time to understand and apply correctly.
How to Tackle OOP in C#
- Learn by Building Projects: Try creating a simple application to learn how classes interact.
- Break Down Each Concept: Focus on one concept (like inheritance) before moving to the next.
3. Managing Memory with Garbage Collection
C# includes automatic garbage collection, which can make memory management easier but also confusing for beginners who are unsure when memory is freed.
Understanding Garbage Collection in C#
- Know When Objects Go Out of Scope: Review how and when C# frees up memory.
- Use the Dispose() Method: For objects that consume large amounts of memory, learn how to use Dispose() to release resources.
Common Coding Errors and How to Solve Them
4. NullReferenceException
The dreaded NullReferenceException is a common error for new C# programmers. It occurs when you try to use an object reference that hasn’t been assigned.
How to Avoid NullReferenceException
- Initialize Variables Properly: Ensure all objects are created before use.
- Use Null-Coalescing Operators: This provides a fallback if an object is null.
5. Confusion with Data Types and Casting
C# has strict data types, and many new programmers struggle with converting between types, especially when working with int, float, string, etc.
Tips for Handling Data Types
- Familiarize Yourself with Data Types: Spend time understanding the common data types and their uses.
- Practice Type Casting: Experiment with casting between types to see how each conversion works.
Mastering Debugging in C#
6. Learning the Visual Studio Debugger
Visual Studio’s debugger is a powerful tool but can be overwhelming at first. Many new programmers aren’t aware of its full capabilities.
Tips for Effective Debugging
- Use Breakpoints: Place breakpoints to analyze your code one step at a time.
- Inspect Variables: Visual Studio lets you view the values of variables during runtime, helping you spot issues faster.
7. Understanding Exceptions and Error Handling
Handling exceptions gracefully is key to making robust C# applications, but it’s often neglected by new programmers who aren’t used to planning for errors.
How to Improve Error Handling Skills
- Use Try-Catch Blocks: Enclose risky code within try-catch to handle exceptions gracefully.
- Understand the Common Exceptions: Spend time learning about frequently occurring exceptions like IndexOutOfRangeException and InvalidOperationException.
Working with C# Libraries and Frameworks
8. Getting Familiar with .NET Framework and .NET Core
C# relies on .NET, a comprehensive framework that adds functionality and tools. However, the vastness of .NET can be overwhelming.
Tips for Learning .NET
- Start with Basics: Focus on understanding the core libraries, like System and Collections.
- Explore the Documentation: Microsoft’s .NET documentation is a great resource for learning.
9. Mastering LINQ (Language Integrated Query)
LINQ allows for easy querying of collections and databases. However, its syntax can be confusing for beginners who are unfamiliar with query expressions.
How to Get Comfortable with LINQ
- Practice with Small Queries: Start with simple collections and queries to understand the basics.
- Use LINQPad: This tool provides a sandbox environment to test LINQ queries in real-time.
Developing Efficient Code and Avoiding Performance Pitfalls
10. Optimizing Code Performance
New programmers often write inefficient code that can slow down applications. Learning how to optimize code is an essential skill in C#.
Simple Ways to Boost Code Efficiency
- Avoid Redundant Loops: Look for areas where loops can be combined or removed.
- Use async and await for Concurrency: This helps manage multiple tasks without blocking the main thread.
11. Managing Dependencies
Dependency management is crucial in larger projects, but new programmers can find it challenging to organize their code effectively.
Tips for Managing Dependencies
- Learn Dependency Injection: C# provides a dependency injection feature, which makes code more modular.
- Use Interfaces: Interfaces allow you to create flexible, interchangeable components.
Understanding Advanced C# Concepts
12. Async and Await
Asynchronous programming is essential for managing multiple tasks, but the async and await keywords can be confusing for beginners.
How to Approach Async Programming
- Understand Task-Based Asynchronous Pattern: Start by learning about the basics of Task and async/await.
- Practice with Simple Async Operations: Try making a small application that fetches data asynchronously.
13. Delegates and Events
Delegates and events allow for more dynamic code but are often challenging to grasp for new C# programmers.
Ways to Learn Delegates and Events
- Start with Examples: Work through examples to understand how delegates and events operate.
- Implement a Simple Event Handler: Try creating a basic event to see how it all fits together.
14. Working with Interfaces and Abstract Classes
Interfaces and abstract classes allow for more structured and organized code. However, knowing when and how to use them can be tricky for beginners.
Best Practices for Using Interfaces and Abstract Classes
- Use Interfaces for Flexibility: Interfaces allow you to switch out components easily.
- Apply Abstract Classes for Shared Code: Use abstract classes when you have code that should be shared across related classes.
Common Pitfalls and How to Avoid Them
15. Overusing Static Methods and Variables
Static methods and variables are convenient but can lead to issues with memory and flexibility if overused.
When to Use Static Elements
- Reserve for Utility Functions: Use static methods for reusable, stateless functions.
- Avoid in Modular Code: Static elements can restrict flexibility in more complex projects.
Conclusion
Getting started with C# presents challenges, but each hurdle comes with valuable learning experiences. From mastering syntax to managing dependencies and understanding complex concepts like async programming, every new skill brings you closer to becoming a confident C# developer. Remember, practice makes perfect, and as you work through these challenges, you’ll build a solid foundation in C#.
FAQs
1. How can I avoid common errors in C#?
Learning to use the Visual Studio debugger and placing breakpoints can help you identify errors early on. Start small, and take time to understand each error message.
2. What’s the best way to understand object-oriented programming in C#?
Begin with small projects that let you explore how classes, objects, and inheritance work. Understanding real-life analogies, like treating classes as blueprints, can help solidify these concepts.
3. How can I improve my coding efficiency in C#?
Learning async programming, optimizing loops, and using efficient data structures are great ways to start. Practice writing clean, modular code.
4. Do I need to learn .NET to be good at C#?
Yes, understanding .NET will give you access to powerful libraries and tools that can significantly enhance your productivity with C#.
5. How do I get comfortable with LINQ in C#?
LINQ can be tricky at first. Start with simple queries, and practice frequently. LINQPad is a helpful tool that lets you experiment with LINQ in a sandbox environment.